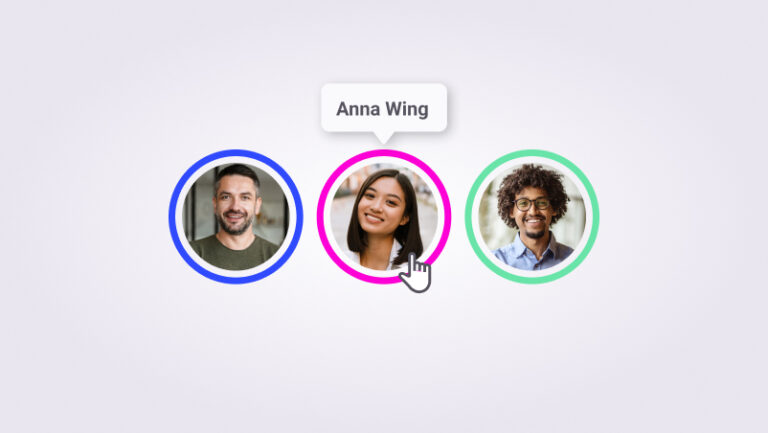
It’s always nice to know you’re not alone, and in a connected and online world, we are seeing tools, like Miro, Notion, Google Docs, making sure that you feel connected to the person you are working on a project with. One of the ways to do that is to display who is online on a page.
It’s quite simple to do that, and in this post, I’m going to show you how to use SuperViz, a powerful collaboration SDK and API, to easily add a Who-is-Online component to your application with just a few lines of code. You’ll learn how to:
- Learn what SuperViz is and how to install the SDK
- Initialize a room with SuperViz and connect your users to it
- Display the online users with avatars, badges, and profile images
What is SuperViz?
SuperViz is a complete collaboration SDK and JavaScript for developers. It allows you to easily add presence awareness, video, and contextual comments to your web app in only a few hours. It provides a flexible and low-code solution for enhancing your web app’s collaborative functionalities.
Install SuperViz SDK
Before you can start using SuperViz, you’ll need to install the @superviz/sdk
package. You can do this using npm or yarn. If you’re using npm, run the following command in your terminal: npm install @superviz/sdk
. If you’re using yarn, the command will be yarn add @superviz/sdk
.
Once the package has been installed, you can import it into your project:
import SuperViz from "@superviz/sdk"
How to initialize a room with SuperViz
The first step to adding a Who-is-Online component to your web page is to initialize a room with SuperViz. A room is a virtual space where your users can join and collaborate. Each room has a unique ID, which you can specify when you create it.
To create a room, you need to use the SuperVizRoom
that’s available from the SDK package, which takes the Developer Key and an object as a parameter. The object should have the following properties:
id
: The ID of the room, which should be a unique string shared between the participants of that room.participant
: An object that contains information about the current user, such asname
,id
.group
: An object that contains information about the group that the user belongs to, such asname
andid
.
Here’s an example of how to create a room with SuperViz:
// Import the SuperViz SDK
import SuperVizRoom from '@superviz/sdk';
// Create a room object
const room = await SuperVizRoom(DEVELOPER_KEY, {
roomId: "",
group: {
id: "",
name: "",
},
participant: {
id: "",
name: ""
},
});
Please note that when you create a room you will need to pass your DEVELOPER_KEY
as a parameter. You can retrieve a free DEVELOPER_KEY
at superviz.com
Once you have created a room, you can use the room
, object to add components, like Who-is-Online.
How to display the online users with SuperViz
The next step is to add the Who-is-Online component to your web page. SuperViz provides a ready-to-use component called WhoIsOnline
, which shows the avatars of people that are currently in the same room, or page, as you.
To add a Who-is-Online component to a specific HTML element on your web page, use the following code:
import { WhoIsOnline } from "@superviz/sdk/lib/components"
const whoIsOnline = new WhoIsOnline();
room.addComponent(whoIsOnline);
When creating the new WhoIsOnline();
you can pass an option to specify the location where the component will be displayed. It can be top-right
, top-left
, bottom-right
, bottom-left
, or an ID of an HTML element.
Here is the full code of adding a Who-is-Online component to your page:
import SuperVizRoom from '@superviz/sdk';
import { WhoIsOnline } from "@superviz/sdk/lib/components"
const room = await SuperVizRoom(DEVELOPER_KEY, {
roomId: "",
group: {
id: "",
name: "",
},
participant: {
id: "",
name: ""
},
});
const whoIsOnline = new WhoIsOnline();
room.addComponent(whoIsOnline);
You can find this code ready to use in the sample’s repository, which provides practical examples of how to use our SDK. Feel free to check it out for further insights and code snippets.
In conclusion, with SuperViz, adding a Who-is-Online component to your website is a straightforward process, which will significantly contribute to enhancing the collaborative experience of your users.
If you have any questions or face any issues while implementing this feature, please feel free to join our Discord server.