SuperViz for Matterport SDK
The missing piece to turn Matterport Tours into a powerful collaboration environment.
See live demoFor developers, by developers
Our straightforward, feature-complete API and SDK integrates into your project with minimal code
1import { RoomProvider, useRoom } from '@superviz/react';2import { Presence3D } from "@superviz/matterport-plugin";3import { VideoConference, WhoIsOnline } from "@superviz/sdk";4import { useCallback, useEffect, useRef, useState } from "react";5import { v4 as generateId } from "uuid";67// Replace with your actual keys8const MATTERPORT_KEY = "YOUR_MATTERPORT_KEY";9const SUPERVIZ_KEY = "sv_YOUR_SUPERVIZ_KEY";10const ROOM_ID = "superviz-demo-matterport";11const MODEL_ID = "Zh14WDtkjdC"; // Replace with your Matterport model ID1213// Type definition for Matterport SDK14type WindowWithMP_SDK = Window & {15MP_SDK: {16connect: (window: Window, key: string) => Promise<any>;17};18};1920function MatterportViewer() {21const containerId = "matterport-container";22const mpSdk = useRef<any | null>(null);23const [sdkReady, setSdkReady] = useState(false);24const participantId = generateId();2526// Use the React SDK's useRoom hook27const { room, joinRoom, leaveRoom, addComponent, removeComponent } = useRoom();2829// Initialize Matterport SDK30const initializeMatterport = useCallback(async () => {31const showcase = document.getElementById(containerId) as HTMLIFrameElement;32if (!showcase) return;3334showcase.onload = async () => {35try {36const showcaseWindow = showcase.contentWindow as WindowWithMP_SDK;37mpSdk.current = await showcaseWindow.MP_SDK.connect(38showcaseWindow,39MATTERPORT_KEY40);41setSdkReady(true);42} catch (error) {43console.error('Failed to initialize Matterport SDK:', error);44}45};46}, []);4748// Initialize SuperViz room and components when SDK is ready49useEffect(() => {50if (!sdkReady || !mpSdk.current) return;5152const handleJoin = async () => {53await joinRoom({54participant: {55id: participantId,56name: "Participant",57avatar: {58imageUrl: 'https://production.cdn.superviz.com/static/default-avatars/1.png',59}60},61group: {62name: 'superviz-demo',63id: 'superviz-demo',64},65roomId: ROOM_ID,66});6768// Create and add the Matterport presence component69const matterportPresence = new Presence3D(mpSdk.current, {70isAvatarsEnabled: true,71isLaserEnabled: true,72isNameEnabled: true,73});7475// Add additional components76const whoIsOnline = new WhoIsOnline({ position: 'bottom-right' });77const videoConference = new VideoConference({78enableFollow: true,79enableGather: true,80enableGoTo: true,81participantType: 'host',82defaultAvatars: true,83});8485addComponent(matterportPresence);86addComponent(whoIsOnline);87addComponent(videoConference);88};8990handleJoin();9192return () => {93leaveRoom();94if (mpSdk.current) {95mpSdk.current.disconnect();96}97};98}, [sdkReady, joinRoom, leaveRoom, addComponent]);99100useEffect(() => {101initializeMatterport();102}, [initializeMatterport]);103104return (105<div className="w-full h-full">106<iframe107className="w-full h-full border-0"108id={containerId}109src={`/mp-bundle/showcase.html?&brand=0&mls=2&mt=0&search=0&kb=0&play=1&qs=1&applicationKey=${MATTERPORT_KEY}&m=${MODEL_ID}`}110allowFullScreen111/>112</div>113);114}115116export function MatterportWithSuperViz() {117return (118<RoomProvider developerToken={SUPERVIZ_KEY}>119<MatterportViewer />120</RoomProvider>121);122}
1import { Presence3D } from "@superviz/matterport-plugin";2import { VideoConference, WhoIsOnline } from "@superviz/sdk";3import { createRoom } from '@superviz/room';4import { ref, onMounted, onBeforeUnmount } from 'vue';5import { v4 as generateId } from "uuid";67// Replace with your actual keys8const MATTERPORT_KEY = "YOUR_MATTERPORT_KEY";9const SUPERVIZ_KEY = "sv_YOUR_SUPERVIZ_KEY";10const ROOM_ID = "superviz-demo-matterport";11const MODEL_ID = "Zh14WDtkjdC"; // Replace with your Matterport model ID1213export default {14setup() {15const containerId = "matterport-container";16const room = ref(null);17const mpSdk = ref(null);18const sdkReady = ref(false);1920async function initializeSuperViz() {21const participantId = generateId();2223room.value = await createRoom({24developerToken: SUPERVIZ_KEY,25roomId: ROOM_ID,26participant: {27name: "Participant",28id: participantId,29avatar: {30imageUrl: 'https://production.cdn.superviz.com/static/default-avatars/1.png',31}32},33group: {34name: "superviz-demo",35id: "superviz-demo",36},37});3839// Create and add the Matterport presence component40const matterportPresence = new Presence3D(mpSdk.value, {41isAvatarsEnabled: true,42isLaserEnabled: true,43isNameEnabled: true,44});4546// Add additional components47const whoIsOnline = new WhoIsOnline({ position: 'bottom-right' });48const videoConference = new VideoConference({49enableFollow: true,50enableGather: true,51enableGoTo: true,52participantType: 'host',53defaultAvatars: true,54});5556room.value.addComponent(matterportPresence);57room.value.addComponent(whoIsOnline);58room.value.addComponent(videoConference);59}6061async function initializeMatterport() {62const showcase = document.getElementById(containerId);63if (!showcase) return;6465showcase.onload = async () => {66try {67const showcaseWindow = showcase.contentWindow;68mpSdk.value = await showcaseWindow.MP_SDK.connect(69showcaseWindow,70MATTERPORT_KEY71);72sdkReady.value = true;73await initializeSuperViz();74} catch (error) {75console.error('Failed to initialize Matterport SDK:', error);76}77};78}7980onMounted(() => {81initializeMatterport();82});8384onBeforeUnmount(() => {85if (room.value) {86room.value.leave();87}88if (mpSdk.value) {89mpSdk.value.disconnect();90}91});9293return {94containerId,95MODEL_ID,96MATTERPORT_KEY97};98},99template: `100<div class="w-full h-full">101<iframe102class="w-full h-full border-0"103:id="containerId"104:src="`/mp-bundle/showcase.html?&brand=0&mls=2&mt=0&search=0&kb=0&play=1&qs=1&applicationKey=${MATTERPORT_KEY}&m=${MODEL_ID}`"105allowFullScreen106/>107</div>108`109};
1import { Presence3D } from "@superviz/matterport-plugin";2import { VideoConference, WhoIsOnline } from "@superviz/sdk";3import { createRoom } from '@superviz/room';4import { v4 as generateId } from "uuid";56// Replace with your actual keys7const MATTERPORT_KEY = "YOUR_MATTERPORT_KEY";8const SUPERVIZ_KEY = "sv_YOUR_SUPERVIZ_KEY";9const ROOM_ID = "superviz-demo-matterport";10const MODEL_ID = "Zh14WDtkjdC"; // Replace with your Matterport model ID11const CONTAINER_ID = "matterport-container";1213let room;14let mpSdk;1516async function initializeSuperViz() {17const participantId = generateId();1819room = await createRoom({20developerToken: SUPERVIZ_KEY,21roomId: ROOM_ID,22participant: {23name: "Participant",24id: participantId,25avatar: {26imageUrl: 'https://production.cdn.superviz.com/static/default-avatars/1.png',27}28},29group: {30name: "superviz-demo",31id: "superviz-demo",32},33});3435// Create and add the Matterport presence component36const matterportPresence = new Presence3D(mpSdk, {37isAvatarsEnabled: true,38isLaserEnabled: true,39isNameEnabled: true,40});4142// Add additional components43const whoIsOnline = new WhoIsOnline({ position: 'bottom-right' });44const videoConference = new VideoConference({45enableFollow: true,46enableGather: true,47enableGoTo: true,48participantType: 'host',49defaultAvatars: true,50});5152room.addComponent(matterportPresence);53room.addComponent(whoIsOnline);54room.addComponent(videoConference);55}5657function initializeMatterport() {58// Create the iframe element59const iframe = document.createElement('iframe');60iframe.id = CONTAINER_ID;61iframe.className = "w-full h-full border-0";62iframe.src = `/mp-bundle/showcase.html?&brand=0&mls=2&mt=0&search=0&kb=0&play=1&qs=1&applicationKey=${MATTERPORT_KEY}&m=${MODEL_ID}`;63iframe.allowFullscreen = true;6465// Append to container66const container = document.getElementById('app');67container.appendChild(iframe);6869// Initialize SDK when iframe loads70iframe.onload = async () => {71try {72const showcaseWindow = iframe.contentWindow;73mpSdk = await showcaseWindow.MP_SDK.connect(74showcaseWindow,75MATTERPORT_KEY76);77await initializeSuperViz();78} catch (error) {79console.error('Failed to initialize Matterport SDK:', error);80}81};82}8384// Clean up function85function cleanup() {86if (room) {87room.leave();88}89if (mpSdk) {90mpSdk.disconnect();91}92}9394// Initialize when the DOM is loaded95document.addEventListener('DOMContentLoaded', initializeMatterport);9697// Clean up when window unloads98window.addEventListener('beforeunload', cleanup);
Testimonials
We have wide experience dealing with Matterport users and we have heard great feedback from our users:
SuperViz turns Matterport into a Multi-Player Metaverse Experience - it is more fun to explore together and it can enhance the team productivity when discussing things virtually on location while leaving a lasting impression.
Florian Wagner
Mesh Images | Germany
The ability to communicate with over 200 guests and up to 16 main speakers is an astonishing educational and collaborative experience. The truth is the use cases are actually beyond our current imagination.
Tony Pincham
Images for Industry Limited | England
Superviz morphs Matterport into something it's not, a collaborative interactive software.
Abe Truitt
Dive Virtual | Israel
Superviz adds value to meetings that are normally dull like Teams or Zoom and you can easily lead attendees through a building to show them something.
Michael de Vries
InformAR | Netherlands
Superviz takes our Matterport service to the next level! First, there was the ability to digitally walk through a building and that still blows minds. Now with Superviz, it has gone to a completely new level and value proposition.
Dean Scholey
VirtualPro Limited | New Zealand
Matterport virtual tours by themselves are a great marketing tool, but Superviz's immersive meetings make them a powerful sales tool.
Emma Urbina
Jotavirtual | Spain
real-time_demo
Real-estate sales tool
Why Choose SuperViz
Create your first proof of concept in minutes! Our tutorials and demos are here to guide you.
Intuitive documentation and a free account - The ideal combination to run your first tests.
We love collaboration! Our Discord community is open for feedback and support.
Get access to a demos and samples to build even faster.
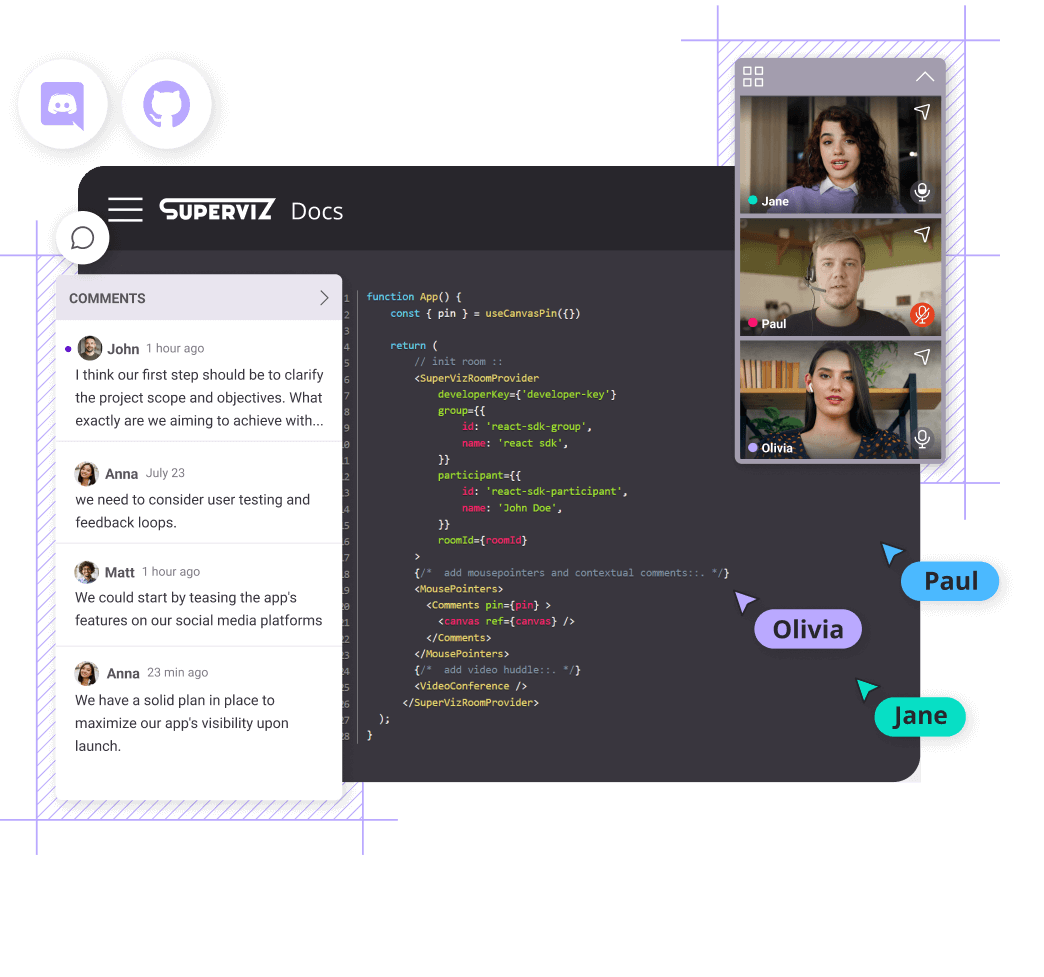
Extend your Matterport platform
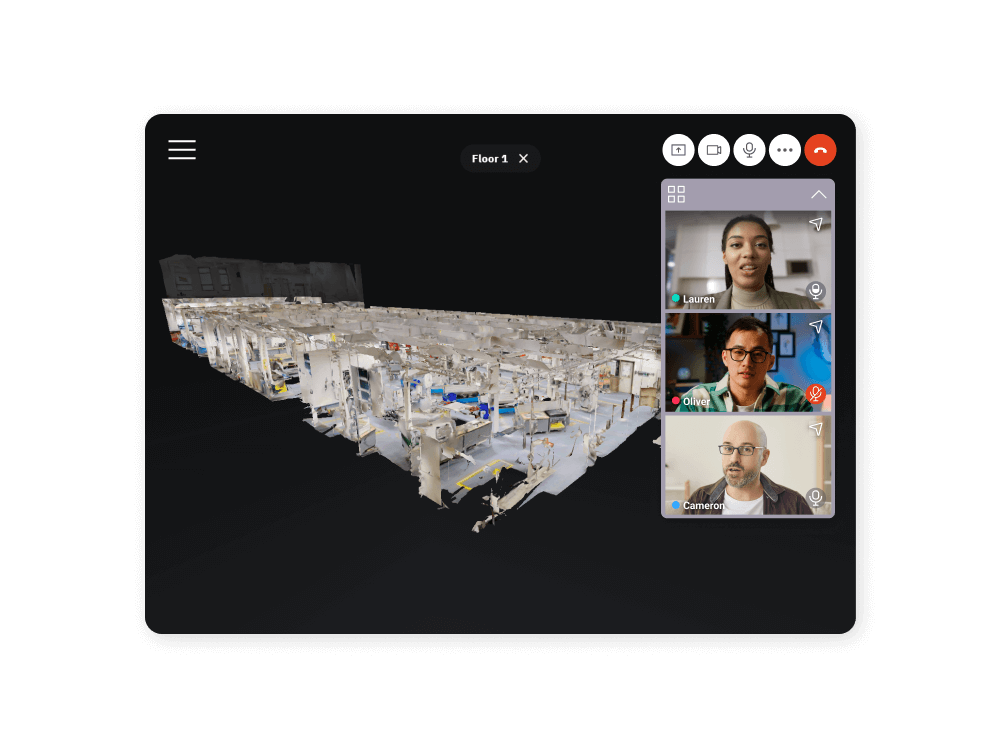
Video Huddle SDK
Integrate high-quality video conferencing directly into your matterport platform, allowing users to connect face-to-face for a virtual guided tours.
Real-time mouse pointers
Real-time Mouse pointers enhance user engagement while exploring the Matterport space among participants.
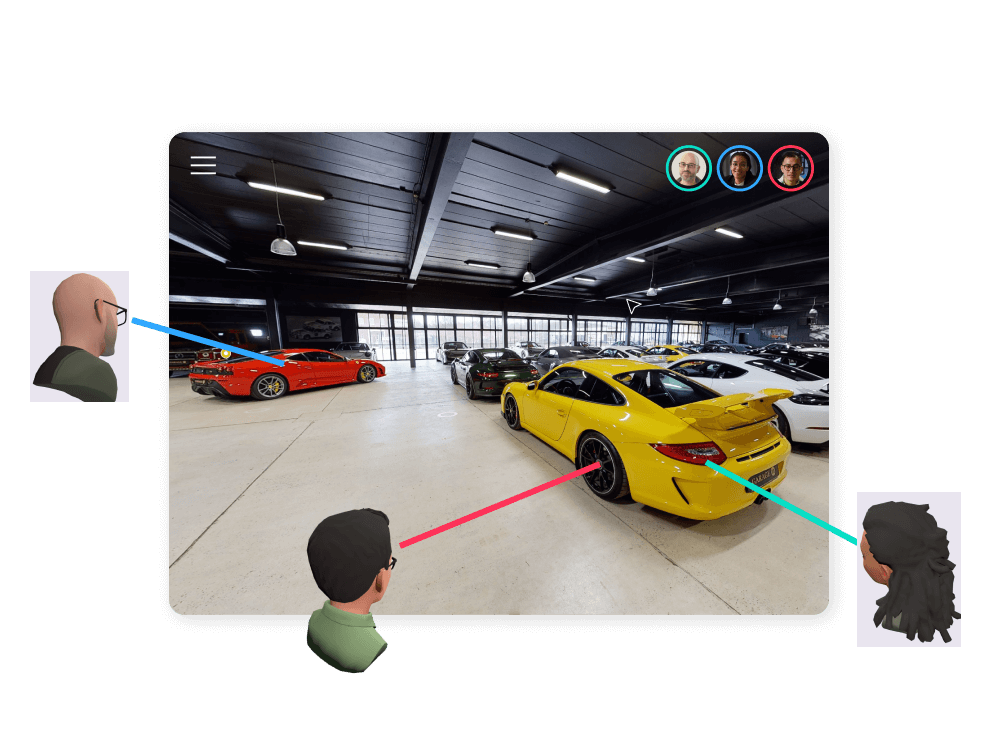
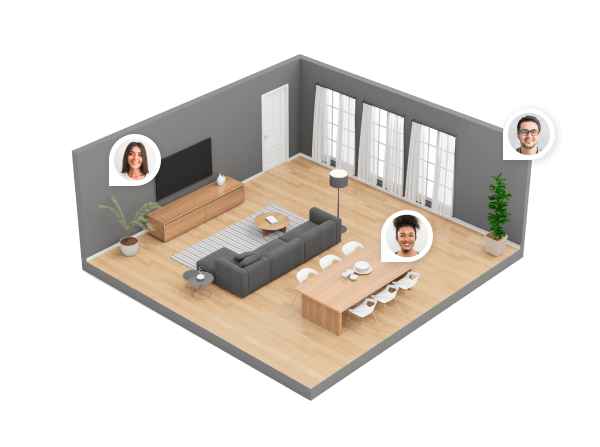
Pinpoint Location inside 3D
Precisely place comments within 3D spaces, ensuring clarity and context in collaborative feedback and discussions.
3D Avatars
3D avatars indicate collaborators' positions and actions, facilitating a better collaborative experience with a sense of presence.
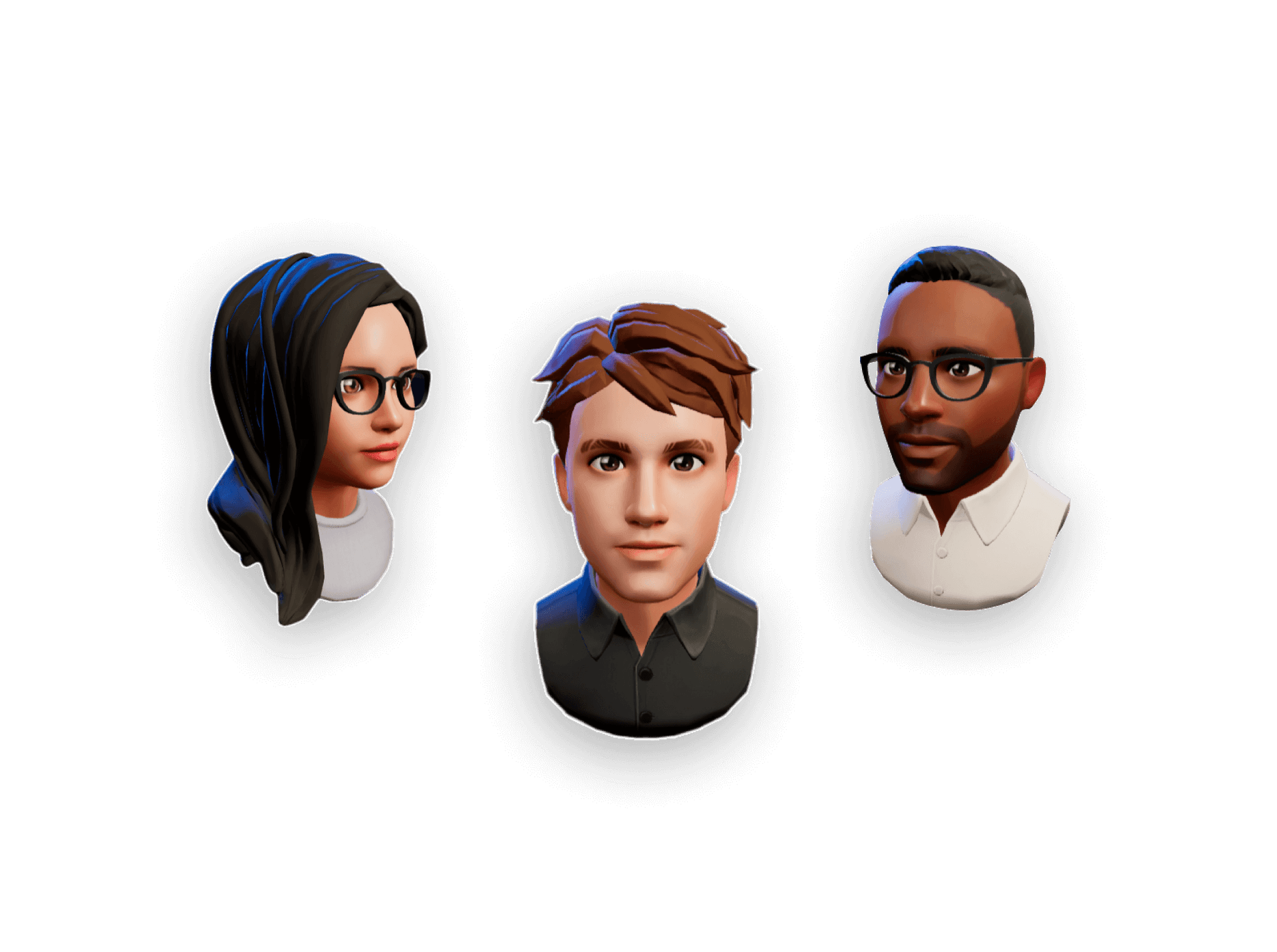
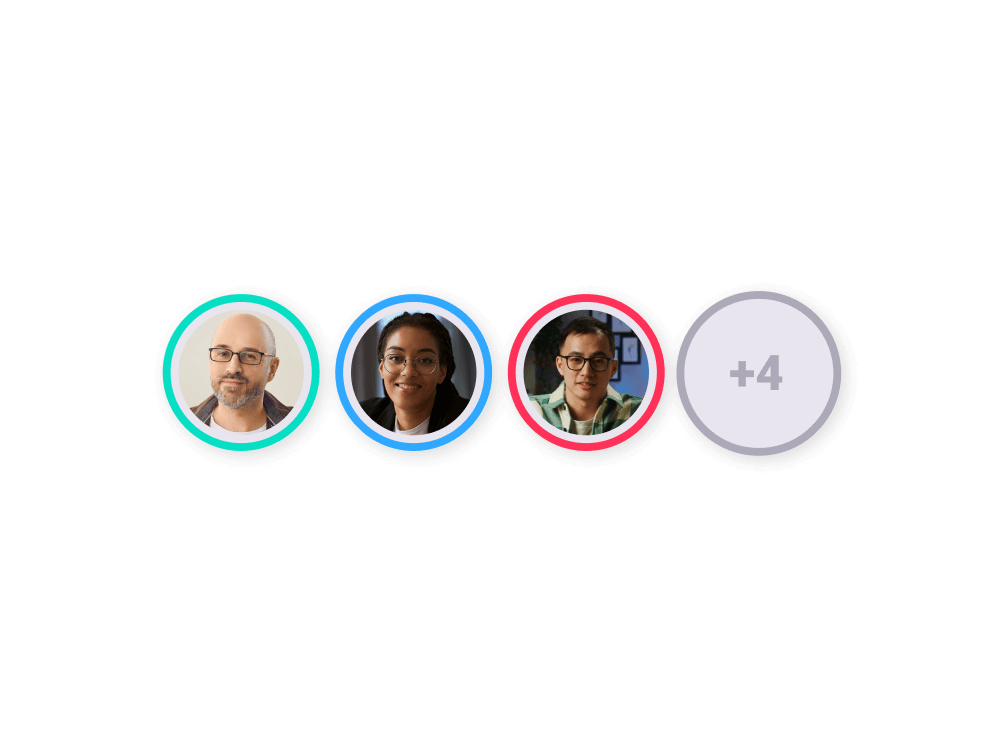
See who's online
Add avatars, badges, profile images to your application to quickly see who is online inside the same room.
AI Meetings Insights
Transcribe and analyze your video conference meetings. Extract key insights, pinpoint action items, track central topics.
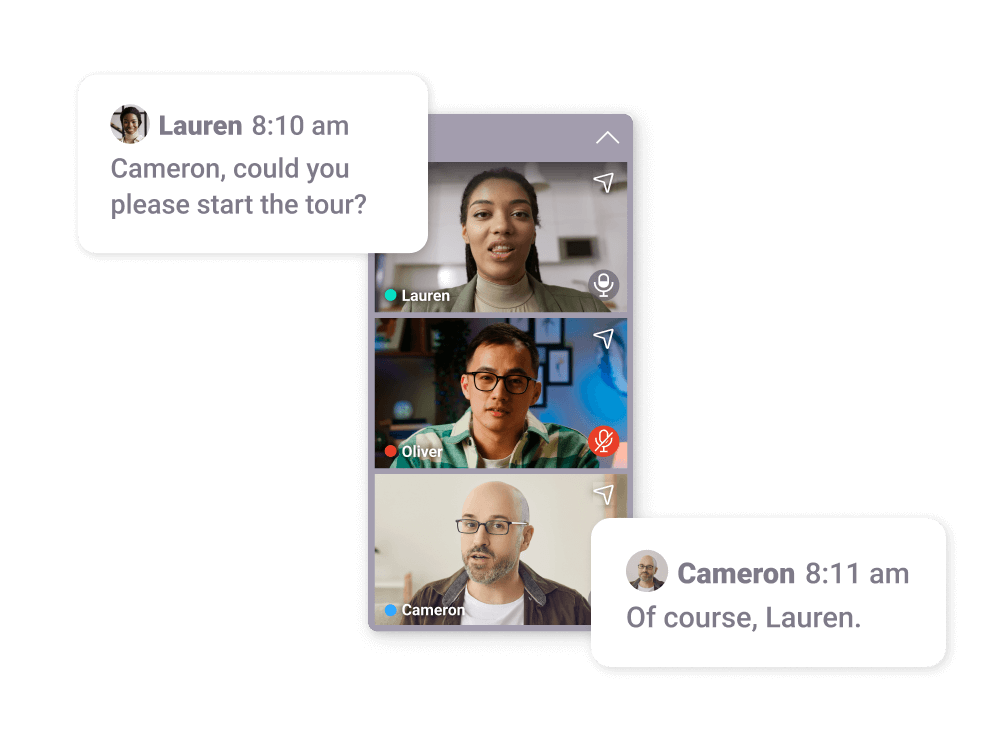
What is SuperViz?
SuperViz offers developers a comprehensive SDK for building interactive, real-time features. Ideal for enhancing design and data workflows, it provides a collection of collaboration components that integrates into modern web-applications.
What is Matterport?
Matterport is a cutting-edge platform that creates immersive 3D virtual tours of physical spaces. It offers a JavaScript Software Development Kit (SDK) that can integrate with web applications and enhance the experience around our WebGL 3D player, Showcase.
This website uses cookies to give you the best experience.